Arrays
An array stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number0, number1, ..., and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and ..., numbers[99] to represent individual variables. A specific element in an array is accessed by an index.
All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
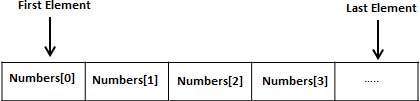
//Source code for C Sharp Arrays
using System;namespace Application_Array
{
class Program_Array
{
static void Main(string[] args)
{
int[] n = new int[10]; /* n is an array of 10 integers */
int i, j;
/* initialize elements of array n */
for (i = 0; i < 10; i++)
{
n[i] = i + 10;
}
/* output each array element's value */
Console.WriteLine("=====OUTPUT=====");
for (j = 0; j < 10; j++)
{
Console.WriteLine("Element[{0}] = {1}", j, n[j]);
}
Console.ReadKey();
}
}
}
OUTPUT
=====OUTPUT=====Element[0] = 10
Element[1] = 11
Element[2] = 12
Element[3] = 13
Element[4] = 14
Element[5] = 15
Element[6] = 16
Element[7] = 17
Element[8] = 18
Element[9] = 19
No comments:
Post a Comment